Hi @Abdou/Fawzy Aboyoussef
using Microsoft.EntityFrameworkCore;
using Microsoft.Extensions.DependencyInjection;
About the above using
reference, since you are using EF core to work with a database, we need to add the EF core related packages: you can right click the project and select the "Manage NuGet Package..." operation, then in the Nuget
panel, you can "Browse" the EF core related package and install them:
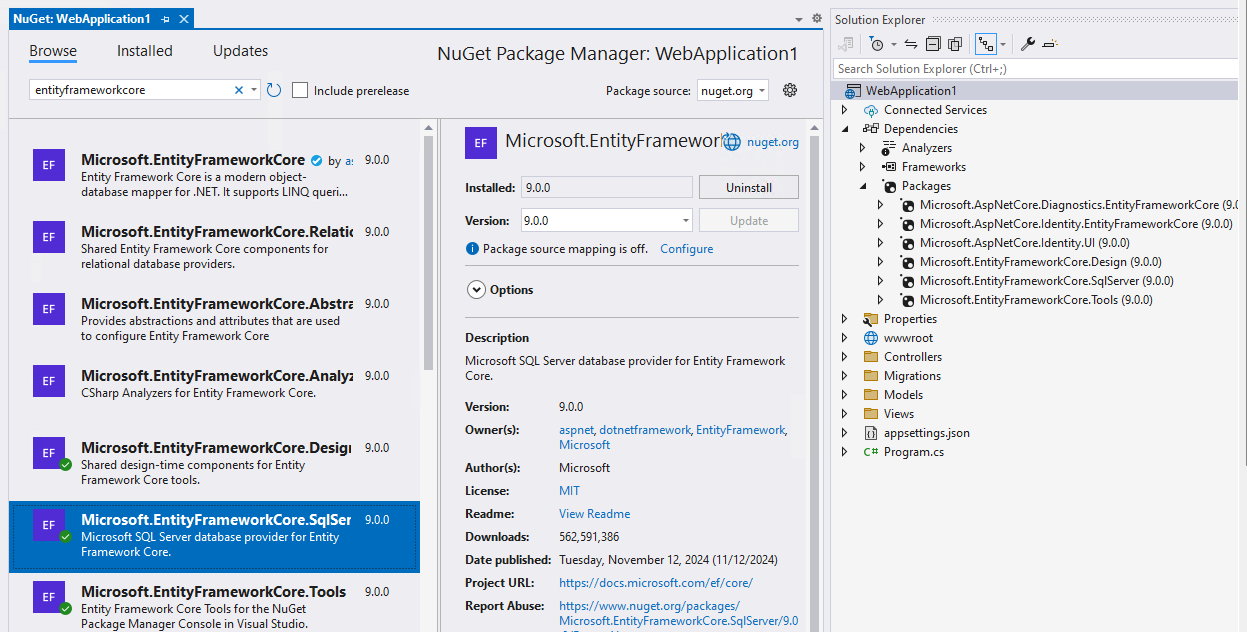
In my sample, I installed the following packages:
<ItemGroup>
<PackageReference Include="Microsoft.AspNetCore.Diagnostics.EntityFrameworkCore" Version="9.0.0" />
<PackageReference Include="Microsoft.AspNetCore.Identity.EntityFrameworkCore" Version="9.0.0" />
<PackageReference Include="Microsoft.AspNetCore.Identity.UI" Version="9.0.0" />
<PackageReference Include="Microsoft.EntityFrameworkCore.Design" Version="9.0.0">
<PrivateAssets>all</PrivateAssets>
<IncludeAssets>runtime; build; native; contentfiles; analyzers; buildtransitive</IncludeAssets>
</PackageReference>
<PackageReference Include="Microsoft.EntityFrameworkCore.SqlServer" Version="9.0.0" />
<PackageReference Include="Microsoft.EntityFrameworkCore.Tools" Version="9.0.0" />
</ItemGroup>
After that, when using the EF Core related methods, you could use the using
statement to add the package reference.
I suggest you could check this tutorial: Get started with ASP.NET Core MVC. And check the links one by one, it contains the detail steps to create MVC application and use EF core:
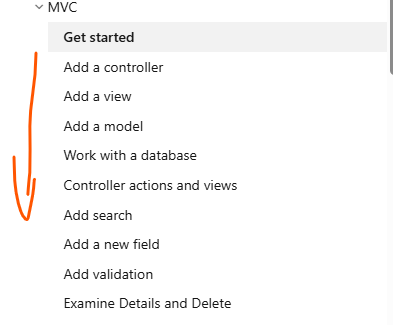
Error (active) CS1061 'WebApplication' does not contain a definition for 'MapStaticAssets'
Besides, about the original question, might be you are not using the default template auto generated Program.cs
file, or you are making a great changing. I suggest you can refer to my reply in this thread:
From .NET 6, the new application template uses the New Hosting Model, it will unify Startup.cs
and Program.cs
into a single Program.cs
file and uses top-level statements to minimize the code required for an app. So we can add services and middleware in the Program.cs
file like this:
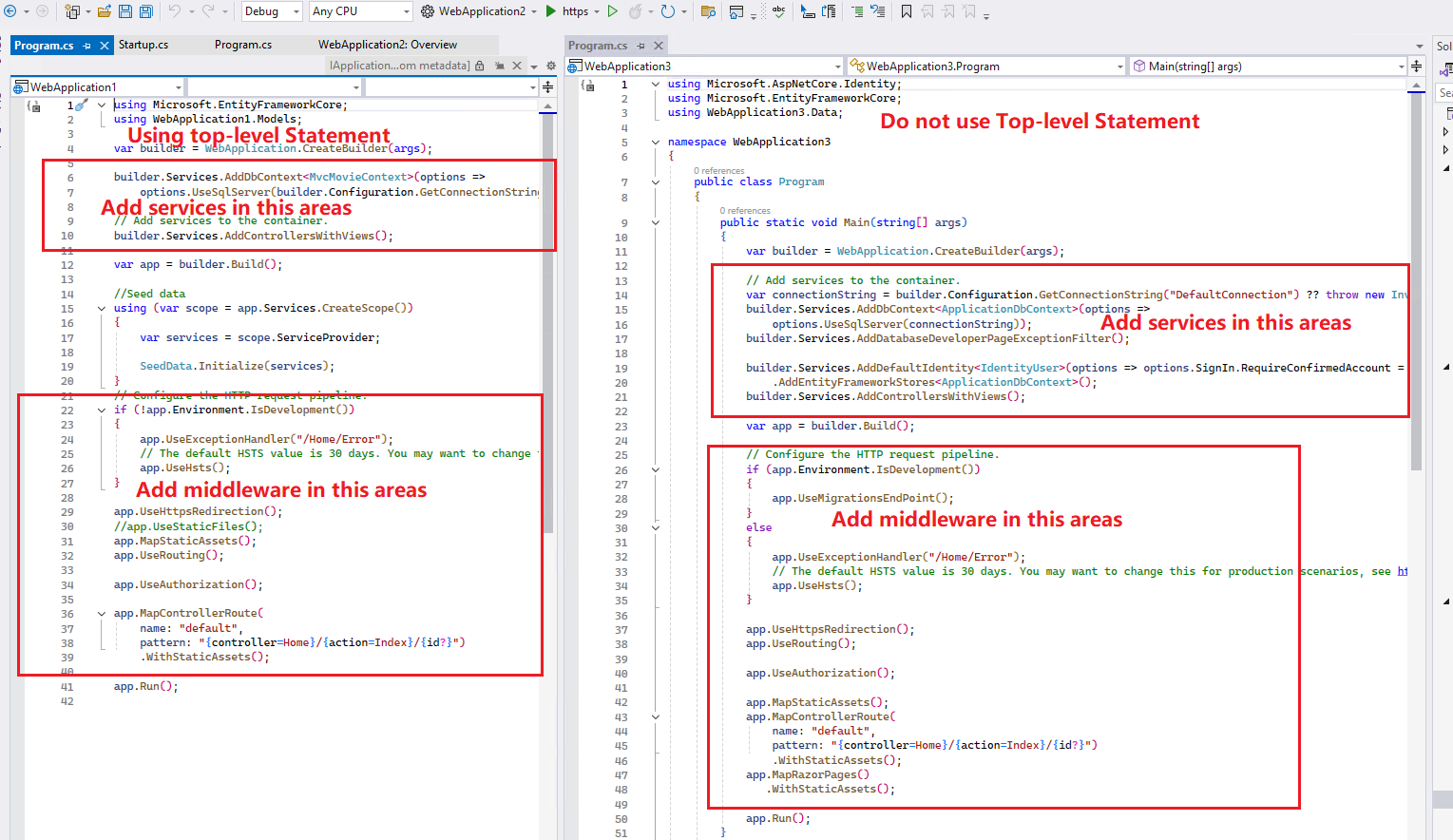
More detail information, see:
New Hosting Model
Code samples migrated to the new minimal hosting model in ASP.NET Core 6.0
If you want to convert the application to use Startup.cs
and Program.cs
file. In the new template application, you can add a Startup.cs
file and refer to the following code:
Program.cs file:
public class Program
{
public static void Main(string[] args)
{
var builder = WebApplication.CreateBuilder(args);
// Set up logging
builder.Logging.ClearProviders();
builder.Logging.AddConsole();
builder.Logging.AddDebug();
// Create a logger factory to pass to Startup
using var loggerFactory = LoggerFactory.Create(logging =>
{
logging.AddConsole();
logging.AddDebug();
});
var logger = loggerFactory.CreateLogger<Startup>();
// Initialize Startup and configure services
var startup = new Startup(builder.Configuration, logger);
startup.ConfigureServices(builder.Services);
var app = builder.Build();
// Configure the middleware pipeline
startup.Configure(app, app.Environment);
app.Run();
}
}
Startup.cs file:
using Microsoft.AspNetCore.Identity;
using Microsoft.EntityFrameworkCore;
using WebApplication2.Data;
namespace WebApplication2
{
public class Startup
{
private readonly IConfiguration Configuration;
private readonly ILogger<Startup> _logger;
public Startup(IConfiguration configuration, ILogger<Startup> logger)
{
Configuration = configuration;
_logger = logger;
// Log a message during startup initialization
_logger.LogInformation("Startup initialized.");
}
public void ConfigureServices(IServiceCollection services)
{
_logger.LogInformation("Configuring services...");
services.AddControllersWithViews();
services.AddRazorPages();
// Add other services here
//// Add services to the container.
var connectionString = Configuration.GetConnectionString("DefaultConnection") ?? throw new InvalidOperationException("Connection string 'DefaultConnection' not found.");
services.AddDbContext<ApplicationDbContext>(options =>
options.UseSqlServer(connectionString));
services.AddDatabaseDeveloperPageExceptionFilter();
services.AddDefaultIdentity<IdentityUser>(options => options.SignIn.RequireConfirmedAccount = true)
.AddEntityFrameworkStores<ApplicationDbContext>();
services.AddControllersWithViews();
}
public void Configure(WebApplication app, IWebHostEnvironment env)
{
_logger.LogInformation("Configuring middleware...");
// Configure the HTTP request pipeline.
if (env.IsDevelopment())
{
app.UseMigrationsEndPoint();
}
else
{
app.UseExceptionHandler("/Home/Error");
// The default HSTS value is 30 days. You may want to change this for production scenarios, see https://aka.ms/aspnetcore-hsts.
app.UseHsts();
}
app.UseHttpsRedirection();
app.UseRouting();
app.UseAuthorization();
app.MapStaticAssets();
app.MapControllerRoute(
name: "default",
pattern: "{controller=Home}/{action=Index}/{id?}")
.WithStaticAssets();
app.MapRazorPages()
.WithStaticAssets();
}
}
}
If the answer is the right solution, please click "Accept Answer" and kindly upvote it. If you have extra questions about this answer, please click "Comment".
Note: Please follow the steps in our documentation to enable e-mail notifications if you want to receive the related email notification for this thread.
Best regards,
Dillion